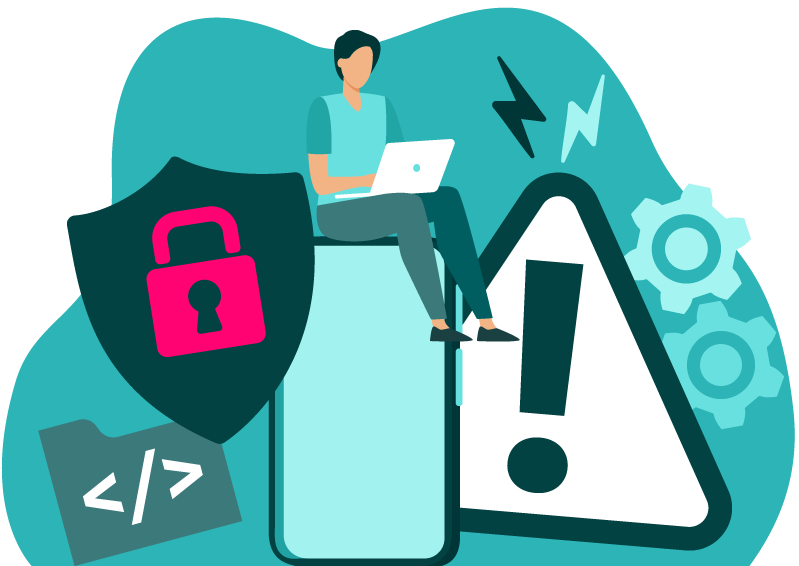
What is CORS Error and When does it Occur?
The CORS (Cross-Origin Resource Sharing) error occurs when a browser blocks an HTTP request to a resource on a domain different from the request's origin. This blocking is a security measure to prevent malicious websites from accessing sensitive resources on other domains.
CORS is a security policy used by browsers to control HTTP requests between different domains. This mechanism is activated in modern browser environments (like Chrome) to protect users from attacks like Cross-Site Scripting (XSS).
Regístrate y prueba enviar SMS con LabsMobile
¡Empieza aquí y ahora!Index
CORS Security Policy
CORS allows servers to specify which origins are permitted to access their resources. It is essential to prevent unauthorized access to protected resources, ensuring that only requests from authorized domains are fulfilled.
Learn more about the CORS Policy.
CORS in SMS API Integrations
The CORS error can arise when attempting to connect with an API, such as LabsMobile's, from a user interface of an application. This problem is common when requests are executed directly from the browser without the proper CORS configuration on the target server.
This happens when a web application tries to make an AJAX request to an API on a different domain without the appropriate permissions configured on the server. This scenario is common in API integrations from user interfaces.
Risks of Direct Browser Connections
Making API calls directly from the browser should be avoided due to security risks. Exposing credentials, such as passwords and API keys, on the client side can result in critical vulnerabilities.
Attackers could intercept this data and use it to access services without authorization. This risk makes the use of secure connections essential.
Designing a Secure Connection with the LabsMobile SMS API
To correctly and securely connect to the LabsMobile API, it is essential to use a backend approach. Instead of making an AJAX call directly from the browser, a request should be made from the application's backend.
The appropriate process involves the browser making a request to the application's backend server, which in turn, makes the secure connection to the LabsMobile API. This method ensures that sensitive credentials are not exposed to the client.
Implementing Secure Connections
To implement a secure connection, the client application should make a call to its own backend. The backend, in turn, should handle authentication and make the request to the LabsMobile API.
For example, in a JavaScript environment, the call from the client to the backend might look like this:
fetch('/api/send-sms', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ message: 'Hello, world!' }) }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
Backend Request to the LabsMobile API
The backend request to the LabsMobile API can be implemented in Python as follows:
import requests def send_sms(message): url = 'https://api.labsmobile.com/sms/send' headers = { 'Authorization': 'Bearer YOUR_API_KEY', 'Content-Type': 'application/json' } payload = { 'to': '1234567890', 'message': message } response = requests.post(url, headers=headers, json=payload) return response.json()
How to Avoid the CORS Error
The CORS error can be avoided by properly configuring HTTP headers on the API server. The Access-Control-Allow-Origin
, Access-Control-Allow-Methods
, and Access-Control-Allow-Headers
headers must be set to allow requests from the desired origins.
This configuration is recommended only in testing or controlled environments due to the aforementioned risks. In production, it is crucial to follow best practices to ensure security.
CORS Header Configuration
To allow CORS requests in a testing environment, the following headers can be added to the web server configuration:
Access-Control-Allow-Origin: http://example.com Access-Control-Allow-Methods: POST, GET, OPTIONS Access-Control-Allow-Headers: Content-Type, Authorization
This configuration allows the server to accept requests from the specified origin, facilitating testing and development.
Conclusion
Understanding and properly managing the CORS error is crucial for API integrations, especially in SMS sending services like LabsMobile. Using a backend approach to make API calls and correctly configuring HTTP headers in controlled environments helps maintain the security and functionality of applications.
For more technical details and support, the LabsMobile team is available to advise and guide through the integration process. Contact us via the chat on our website or through the contact form. This assistance is essential to ensure that applications function securely and efficiently, without compromising data integrity.

Our team advises you
Interested in our services?
Our managers and technical team are always available to answer all your questions about our SMS solutions and to advise you on the implementation of any action or campaign.
Contact us